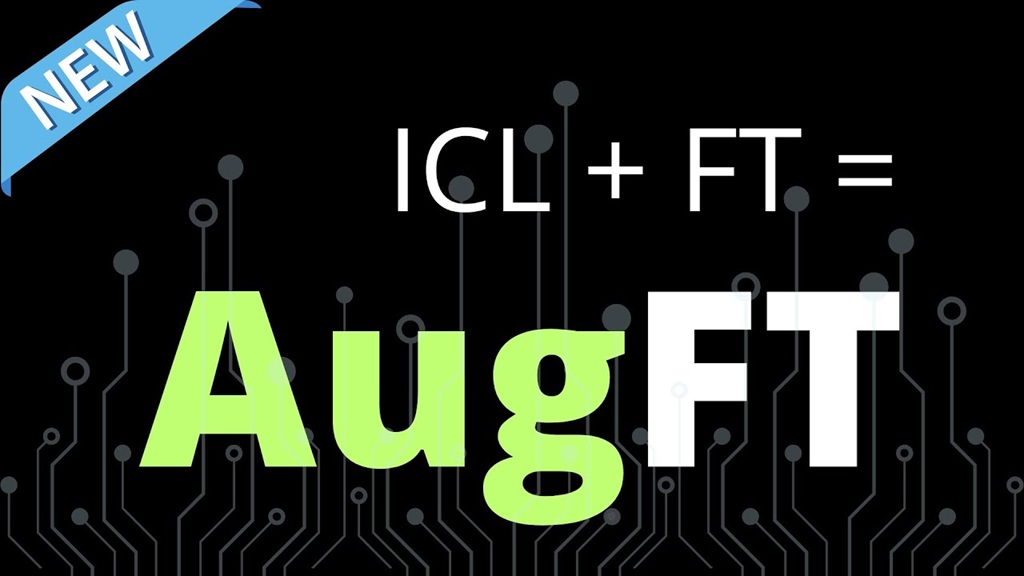
Introduction
This article discusses a groundbreaking approach to fine-tuning Large Language Models (LLMs) that significantly improves their reasoning capabilities. The presenter highlights a fundamental issue with traditional fine-tuning methods: while LLMs can perform logical reasoning tasks like reversals and syllogisms in in-context learning (ICL) mode, they often fail at these same tasks after standard fine-tuning. The video introduces a novel solution that combines the strengths of both approaches.
Why and How: A Smarter Way to Fine-Tune LLMs
Why a Smarter Fine-Tuning Approach is Needed
Traditional fine-tuning of Large Language Models (LLMs) has a significant limitation: it teaches models to memorize specific patterns rather than understand underlying logical relationships. This results in models that cannot generalize well to variations of tasks they were trained on, particularly reasoning tasks like logical reversals (if A→B, then B→A) and syllogisms.
The problem stems from how standard fine-tuning modifies the model’s weights based on specific examples without ensuring the model truly understands the logical principles behind those examples. This leads to brittle performance when the model encounters slight variations of the training data.
How the Smarter Fine-Tuning Works
The solution combines the strengths of in-context learning (ICL) and fine-tuning through a simple but powerful data augmentation technique:
- Start with original training data: Begin with your standard fine-tuning dataset.
- Leverage in-context learning: Feed this data to a capable LLM (typically 7B+ parameters) in ICL mode, where it can perform logical reasoning.
- Generate reasoning examples: Ask the model to perform the desired reasoning tasks (reversals, syllogisms, etc.) based on the original data.
- Augment the dataset: Add these ICL-generated examples to the original training data.
- Fine-tune on augmented data: Fine-tune the model on this expanded dataset that now explicitly includes examples of the desired reasoning patterns.
This approach essentially uses the model’s own ICL capabilities to teach its fine-tuned version how to reason properly. The augmented dataset forces the fine-tuning process to learn the generalization patterns directly, rather than just memorizing specific examples.
Benefits of This Approach
The augmented fine-tuning method provides several advantages:
- Improved reasoning: Models gain the ability to perform logical operations they would otherwise fail at with standard fine-tuning.
- Preserved fine-tuning benefits: The approach maintains the efficiency and deployment benefits of fine-tuning while adding ICL’s flexibility.
- Superior performance: Research shows augmented fine-tuning can match or even exceed pure ICL performance on reasoning tasks.
- Self-improvement: The technique leverages the model’s own capabilities to enhance itself, creating a virtuous cycle of improvement.
This smarter fine-tuning approach represents an important step toward LLMs that don’t just memorize patterns but truly understand logical relationships, making them more reliable for tasks requiring reasoning and generalization.
LLM Augmented Fine-Tuning Implementation Example
Here’s a code example demonstrating how to implement the augmented fine-tuning approach:
import torch
from transformers import AutoModelForCausalLM, AutoTokenizer, TrainingArguments, Trainer
import pandas as pd
def main():
# Step 1: Load a pre-trained LLM
model_name = "gpt2-large" # You might use a larger model in practice
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModelForCausalLM.from_pretrained(model_name)
# Step 2: Prepare your original fine-tuning dataset
original_data = pd.read_csv("original_training_data.csv")
# Step 3: Use the same model in ICL mode to generate augmented examples
augmented_examples = generate_augmented_examples(model, tokenizer, original_data)
# Step 4: Combine original and augmented datasets
combined_data = pd.concat([original_data, augmented_examples])
# Step 5: Fine-tune on the combined dataset
train_dataset = prepare_dataset(combined_data, tokenizer)
# Configure training
training_args = TrainingArguments(
output_dir="./augmented_finetuned_model",
per_device_train_batch_size=4,
num_train_epochs=3,
save_steps=1000,
save_total_limit=2,
learning_rate=5e-5,
)
# Initialize trainer and train
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
)
trainer.train()
# Save the augmented fine-tuned model
model.save_pretrained("./augmented_finetuned_model")
tokenizer.save_pretrained("./augmented_finetuned_model")
def generate_augmented_examples(model, tokenizer, original_data):
"""
Use the model in ICL mode to generate logical variations of original examples
"""
augmented_examples = []
for _, row in original_data.iterrows():
# Extract the original fact or premise
original_fact = row["fact"]
# Create prompts asking for reversals and logical deductions
reversal_prompt = f"""
Based on the following fact, generate its logical reversal:
Fact: {original_fact}
Reversal:
"""
syllogism_prompt = f"""
Based on the following premise, generate a logical conclusion:
Premise: {original_fact}
Conclusion:
"""
# Generate completion using the model in ICL mode
reversal = generate_completion(model, tokenizer, reversal_prompt)
conclusion = generate_completion(model, tokenizer, syllogism_prompt)
# Add generated examples to augmented dataset
augmented_examples.append({
"fact": reversal,
"type": "reversal"
})
augmented_examples.append({
"fact": conclusion,
"type": "syllogism"
})
return pd.DataFrame(augmented_examples)
def generate_completion(model, tokenizer, prompt, max_length=50):
"""Generate text completion using the model"""
inputs = tokenizer(prompt, return_tensors="pt")
# Run in inference mode (no gradient calculation)
with torch.no_grad():
output = model.generate(
inputs["input_ids"],
max_length=len(inputs["input_ids"][0]) + max_length,
temperature=0.7,
top_p=0.9,
do_sample=True,
)
# Decode and return only the newly generated text
generated_text = tokenizer.decode(output[0][len(inputs["input_ids"][0]):], skip_special_tokens=True)
return generated_text.strip()
def prepare_dataset(data, tokenizer):
"""Convert dataframe to format expected by HuggingFace Trainer"""
# Implementation depends on your specific data format
# This is just a placeholder - you would need to implement proper tokenization
pass
if __name__ == "__main__":
main()
This code demonstrates the key concept:
- Start with your original fine-tuning dataset
- Use the model’s own in-context learning capabilities to generate logical variations (reversals and syllogisms)
- Combine the original and generated examples
- Fine-tune on this augmented dataset
In a real implementation, we need to:
- Use a more powerful model (7B+ parameters as mentioned in the video)
- Implement proper data preprocessing and tokenization
- Add evaluation metrics to measure reasoning capability
- Possibly use parameter-efficient fine-tuning methods like LoRA
The core innovation is using the model’s own ICL capabilities to generate examples that force the fine-tuned model to learn logical reasoning patterns rather than just memorizing specific examples.
Video :
Related Section of Video Content
The Problem with Traditional Fine-Tuning
Standard fine-tuning causes LLMs to learn examples too literally, focusing on surface patterns rather than understanding the underlying logic. For example, LLMs fine-tuned on “A is B” statements often fail to recognize “B is A” reversals, despite being capable of handling such reasoning in ICL mode.
The Proposed Solution
The solution leverages the LLM’s own in-context learning capabilities to generate examples of the desired reasoning patterns. The process works as follows:
- Take the original fine-tuning dataset
- Feed this data as context to a powerful base LLM
- Ask the LLM to perform reasoning tasks (like reversals or syllogisms) based on this data
- Collect the generated examples and add them to the original training data
- Fine-tune the model on this augmented dataset
Research Findings
A 2025 study by Google DeepMind and Stanford University demonstrated that this augmented fine-tuning approach dramatically improves performance. While standard fine-tuning showed 0% accuracy on reversal tasks, the augmented fine-tuning method achieved performance comparable to or even surpassing ICL performance. This held true across different reasoning tasks including reversals and syllogistic inferences.
Conclusion
The research reveals that standard fine-tuning often fails because it learns data too rigidly without generalizing to logical variations. The new augmented fine-tuning approach effectively bridges this gap by leveraging the model’s own in-context reasoning abilities to generate explicit examples of these variations, then incorporating them into the fine-tuning dataset. This forces the model to learn generalization patterns directly, resulting in much better reasoning capabilities.
5 Key Takeaways:
- Standard fine-tuning causes LLMs to encode information rigidly, optimizing for predicting exact training sequences rather than understanding underlying logic.
- In-context learning (ICL) operates more dynamically, building temporal knowledge representations that support flexible reasoning like reversals and syllogisms.
- The innovative solution uses an LLM’s own ICL capabilities to generate examples of desired reasoning patterns, then incorporates these into the fine-tuning dataset.
- Tests show the augmented fine-tuning approach can match or exceed ICL performance on reasoning tasks while maintaining the benefits of traditional fine-tuning.
- Smaller LLMs (below 7 billion parameters) showed less ICL benefit, suggesting a minimum model size threshold is needed to leverage this technique effectively.